No love for boolean parameters
— JavaScript, TypeScript, ReactJs — 4 min read
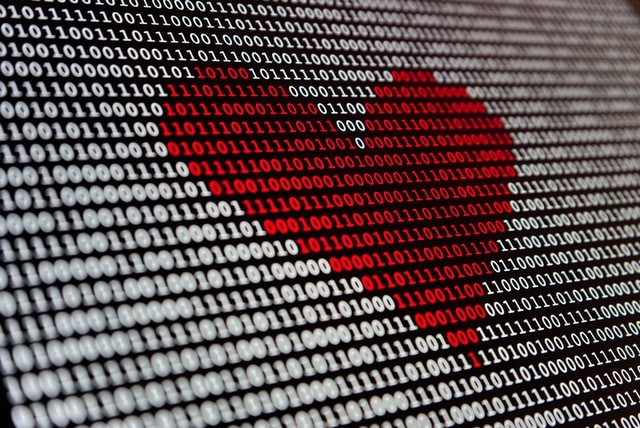
Ah, booleans. 0 or 1, true or false. Always either one of them, never something in between. So simple and predictable. At the end of the day, all code we write winds up in lots of zeros and ones.
There is nothing wrong with booleans per se. We use them for conditions every day:
1// ✅ boolean condition2if (user.age() < legalAge) {3 return 'Sorry, you are too young to use this service'4}
But using them for parameters to a function can be an indication of bad design for various reasons:
Single responsibility principle
A function should do one thing, and one thing only. Passing a "flag" to it often indicates that a function does two things at once, thus violating this principle. For example:
1// 🚨 booleans as a function parameter2function createReport(user: User, sendEmail: boolean) {3 // create the report here4 const reportData = ...5
6 if (sendEmail) {7 sendReport(user.email, reportData)8 }9 return reportData10}
There seem to be some cases where we want to send the report as email directly after creating it, and some cases where we don't. But why entangle this in the createReport function? That function should only create the report, and nothing else. The caller can decide what they want to do with it.
They are confusing
Flags can be confusing, especially in languages where you don't have named parameters. Take for example this signature of equals from the Kotlin standard library:
1fun String?.equals(other: String?, ignoreCase: Boolean): Boolean2
3// Returns true if this string is equal to other, optionally ignoring character case.
As opposed to the first example, the function doesn't do two things at once, it does one thing in two different variations - an important difference. This can be highly confusing when you have to read the call-side that looks something like this:
1"foo".equals("bar", true)2"foo".equals("bar", false)
How should we know what true
means in this context. Even worse, what would false
mean? Does it maybe negate the equals comparison? Scala has solved this differently with two methods: equals and equalsIgnoreCase. Each does one only one thing - no guesswork here.
More guesswork
Before you look it up here - what do you think this boolean flag on Groovy's List.sort
method means:
1["hello","hi","hey"].sort(false) { it.length() }
In case it isn't obvious to everyone:
mutate
- false will always cause a new list to be created, true will mutate lists in place
Totally logical and intuitive api, not confusing at all 🤷♂️
Impossible states
Booleans make it easy to create impossible states. Suppose you have a metric of some sorts, and you want to format that. It might be a "normal" number, but it might also be a percentage value. So you decide to model the formatting function like this:
1function formatMetric(value: number, isPercent: boolean): string {2 if (isPercent) {3 return `${value * 100}%`4 }5 return String(metric)6}
This is rather rudimentary number formatting function, but apart from that, it doesn't look too bad. Frankly, the first "flag" you add to a function usually looks very innocent.
The second flag
Requirements change over time (as they tend to do), and now we have to support currencies for some of our metrics as well. Starting from the above formatting function, we are tempted to add another flag, isCurrency
1function formatMetric(2 value: number,3 isPercent: boolean,4 isCurrency: boolean5): string {6 if (isPercent) {7 return `${value * 100}%`8 }9 if (isCurrency) {10 return // imagine some currency formatting is returned here11 }12 return String(metric)13}
Our code works, we write tests, add the currency flag if we have a currency metric, and all is well.
Except it isn't.
Adding one boolean doesn't add one more state - the amount of states grow exponentially. Two booleans means four states, three booleans means eight possible states etc. What happens if we call our above function with:
1formatMetric(100, true, true)
The answer is: you can't know. It's an implementation detail which flag is checked first. It's also an impossible state: A metric cannot be percent and currency at the same time. Such impossible states are frequently introduced with boolean parameters. I recently encountered a function with 8 booleans as input - turns out, it only had 3 actual states, the rest were variations thereof.
Resist the urge
To avoid impossible states, resist the urge of adding the first boolean parameter. It is infinitely easier for humans to extend existing patterns instead of recognizing anti-patterns and refactoring them. If there is one boolean, there will be a second. If we start of with an enumeration of possible states, it is much more likely that this will be extended instead:
1function formatMetric(value: number, variant?: 'percent'): string {2 if (variant === 'percent') {3 return `${value * 100}%`4 }5 return String(metric)6}
Now we can extend the variant to 'percent' | 'currency'
, and only have three states to work with instead of four. Of course, you can also explicitly include the default (standard) variant instead of using undefined.
Moar advantages
Further advantages of a single variant property include:
Better type safety
We've already covered readability, but it's also very easy to mix flags up, and because they have the same type (boolean), the compiler won't tell you about it. You can work around this by using a single options object, which is quite popular in JavaScript.Exhaustive matching
I've written about exhaustive matching in TypeScript before, and it also comes in very handy in this example. The compiler will then tell us where we need to adapt our code when we add a new variant. CDD, compiler-driven-development:
1type MetricVariant = 'standard' | 'percent' | 'currency'2function formatMetric(3 value: number,4 variant: MetricVariant = 'standard'5): string {6 switch (variant) {7 case 'percent':8 return `${value * 100}%`9 case 'currency':10 return // imagine some currency formatting is returned here11 case 'standard':12 return String(metric)13 }14}
We also do the same when creating React components, or have you seen a Button with an isPrimary and isSecondary flag? Of course not - because how can they be both at the same time?
1// 🚨 Don't do this2<Button isPrimary isSecondary />3
4// ✅ Do this5<Button variant="primary" />
The wrong abstraction
Oftentimes, flags are added because we see similarities to existing code, and we don't want to repeat ourselves, keeping everything DRY.
- Here is a function that looks almost like what I want, I just need to add this one flag, because it's slightly different.
- This component looks like I could use it for my case as well, I just need a withPadding property added so that it fits.
There is lots of good literature available on that topic, showing why we shouldn't do this and what we could do instead:
I can recommend them all, and for starters, resist the urge of adding the next boolean parameter to your codebase.
No matter if you like booleans, or not, or both at the same time, leave a comment below. ⬇️
